Ways to Center a Div or Text in a Div in CSS
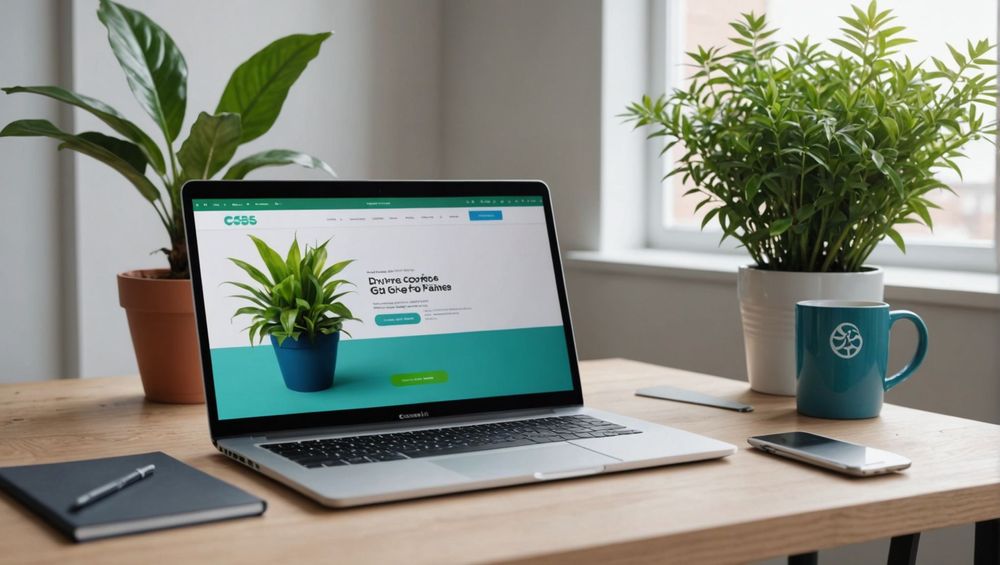
Centering a div or text in a div is a common task when designing web pages, and fortunately, CSS offers multiple ways to achieve this. Whether one is a novice or an experienced developer, knowing a variety of techniques can be extremely useful. In this article, we will explore different methods to center a div or text inside another div using CSS.
Using Flexbox
Flexbox is a CSS layout module designed to create complex layouts with simple and clean code. Centering a div or text using Flexbox is straightforward and highly effective. To center elements with Flexbox, you need to set the parent container to display as flex and use the justify-content and align-items properties.
- First, set the display property of the parent div to flex.
- Use justify-content: center to center the div horizontally.
- Use align-items: center to center the div vertically.
Here is an example:
.parent-div {
display: flex;
justify-content: center;
align-items: center;
height: 100vh; /* Example height */
}
.child-div {
width: 50%;
height: 50%;
background-color: lightcoral;
}
Using Grid Layout
Another powerful tool in CSS is the Grid Layout module, which is even more flexible than Flexbox for two-dimensional layouts. Centering a div within a grid container requires a few straightforward steps:
- Set the display property of the parent div to grid.
- Use place-items: center to center the div both horizontally and vertically.
Here is an example:
.parent-div {
display: grid;
place-items: center;
height: 100vh; /* Example height */
}
.child-div {
width: 50%;
height: 50%;
background-color: lightblue;
}
Using Margin Auto
For situations where you want to horizontally center a block-level element, you can use margin auto. This method is simple and quite effective but only centers elements horizontally.
- Set the width of the child div.
- Use margin: 0 auto to center the div horizontally within its parent.
Here is an example:
.parent-div {
text-align: center; /* To center text inside the child */
}
.child-div {
width: 50%; /* Example width */
margin: 0 auto;
background-color: lightgreen;
}
Using CSS Positioning
This method involves positioning the child div absolutely within the parent container. To center a child div both horizontally and vertically using absolute positioning:
- Set position: relative to the parent div.
- Set position: absolute to the child div.
- Use top, left, transform properties to center the child div.
Here is an example:
.parent-div {
position: relative;
height: 100vh; /* Example height */
}
.child-div {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 50%; /* Example width */
height: 50%; /* Example height */
background-color: lightyellow;
}
Using Text Alignment for Centering Text
If your goal is to center text within a div, text-align property is the easiest way to do so. This method is perfect for inline and inline-block elements:
- Set the text-align property of the parent div to center.
Here is an example:
.parent-div {
text-align: center;
height: 100vh; /* Example height */
background-color: lightgrey;
}
.child-div {
display: inline-block; /* Makes the child inline-block element */
width: 50%;
background-color: white;
}
Conclusion
As demonstrated, there are multiple effective methods for centering a div or text within another div using CSS. Each approach has its strengths and is appropriate in different situations. Whether using modern CSS modules like Flexbox and Grid, or more traditional techniques like margin auto and absolute positioning, you have a robust toolkit to create visually appealing, centered layouts.
FAQ
1. Can I use multiple methods at the same time?
It’s not usually recommended to use multiple centering methods simultaneously as it could lead to unexpected results. Choose the technique that best fits your design requirements.
2. Is Flexbox better than Grid for centering elements?
Flexbox is generally easier for one-dimensional layouts (row or column), while Grid is preferable for complex two-dimensional layouts. Each has its advantages depending on the use case.
3. Does margin auto work for vertical centering?
No, margin auto only centers elements horizontally. For vertical centering, consider using Flexbox, Grid, or absolute positioning.
4. Is it necessary to set the height for centering with Flexbox and Grid?
Yes, setting a height (often 100vh or a specific pixel height) helps to center the child elements correctly within the Flexbox or Grid container.
5. Can I center an image inside a div using these methods?
Yes, images can be centered using the same methods discussed above. Just apply the CSS properties to the parent and child divs containing the image.